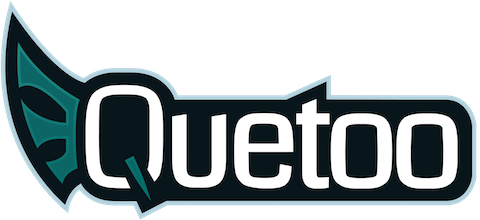
The materials system provides a flexible framework to enhance your levels with animations, light flares, environment maps, terrain blending, and other effects. If you are familiar with Quake 3 Arena's "shaders" system, you'll be right at home with materials. If you're just curious about the basics, and using the in-game materials editor, then this video will get you started:
A material is a set of directives and parameters for an individual world texture. Each texture in your level can have zero or one material definition. Materials are defined in a plain text file called materials/mymap.mat
, where mymap
is the name of your .bsp
file. The BSP compiler can generate a default materials file for your level to get you started: q2wmap -mat maps/my.map
Materials are comprised of a material definition, and then zero or more layers called stages. Each stage can describe one or more effect, and each effect is blended with the result of the prior stages. The basic structure of a materials file is:
{
# Material definition
material path/to/texture1
parameters
{
# Stage definition
texture path/to/texture2
parameters
}
...
}
The material definition specifies the texture name and per-pixel lighting parameters.
${texture}_nm
and ${texture}_norm
. Typically, this directive is omitted. However, if you have several diffuse textures that differ only in color, you can save some texture memory by specifying the same normalmap map for each of them. See normalmaps and bump mapping${texture}_s
and ${texture}_gloss
. Typically, this directive is omitted. However, if you have several diffuse textures that differ only in color, you can save some texture memory by specifying the same glossmap for each of them. See normalmaps and bump mappingb
parameter is required, and must be a positive floating point value, or 0.0
. The default value is 1.0
.1.0
requires high-quality normalmaps, with properly encoded height values. The p
parameter is required, and must be a positive floating point value, or 0.0
. The default value is 1.0
.h
parameter is required, and must be a positive floating point value, or 0.0
. The default value is 1.0
.s
parameter is required, and must be a positive floating point value, or 0.0
. The default value is 1.0
.It is a best practice to define all material parameters for your opaque world surfaces, as they are eligible for per-pixel lighting. Quetoo provides safe defaults when you omit these parameters, but tuning them will often benefit the appearance of your level.
{
material evil6/stone_floor
bump 3.0
hardness 1.5
parallax 1.2
specular 3.0
}
Note that blended materials such as glass or foliage do not support per-pixel lighting, and so you may safely omit the material parameters for them.
{
material office/glass
{
envmap office/glass
}
}
There are four types of stages: texture envmap lightmap flare
. A stage must start with a type declaration, e.g. texture lunaran/pad1_fx
, envmap 0
, lightmap
, flare 1
, etc..
Texture stages are the most versatile and complex type, and must always declare a valid texture image, e.g. texture lunaran/pad1_fx
. The following listing describes the texture stage directives.
1
, e.g. lunaran/computer1
, with subsequent frames following in sequence. The frames
parameter specifies the number of textures which comprise the animation. The hz
parameter specifies the frequency of the effect. Both are required, and must be a positive integer and positive floating point number respectively.src
and dest
parameters are GL constants, e.g. blend GL_ONE GL_SRC_ALPHA
. The default blend function is GL_ONE GL_ONE_MINUS_SRC_ALPHA
.r g b
are each required, and must each be floating point numbers between 0.0
and 1.0
.hz
parameter specifies the frequency of the effect. It is required and must be a positive floating point number.hz
parameter specifies the speed of the rotation. It is required, and must be a positive floating point number.amp
parameter specifies the scale of the stretch, while hz
specifies the frequency. Both are required, and must be positive floating point numbers.ceil
and floor
parameters specify the highest and lowest Z-axis coordinates where blending will occur. At Z coordinates less than floor
, the base material texture will appear; at Z coordinates greater than ceil
, the stage texture will appear. Linear interpolation is used to blend the two textures at Z coordinates between ceil
and floor
. Both parameters are required, and must be floating point numbers.intensity
parameter specifies the overall amplification of the effect. It is required, and must be between 0.0
and 1.0
.Both terrain and dirtmap stages support per-pixel lighting, thus it is possible to create bumpmapped terrain. To do this, declare your terrain stage texture with a material definition prior to the base material.
{
material organics/grass
...
}
{
material organics/dirt
...
{
texture organics/grass
terrain 32.0 128.0
}
}
Environment map stages are used to simulate reflections on metal, water, glass, etc. For convenience, they may be declared with numeric values to take advantage of several built-in effect textures, e.g. envmap 0
. Alternatively, a unique texture can also be provided, e.g. envmap envmaps/my_envmap
.
Environment map stages do not currently support any unique directives, but do support blend color scroll.s scroll.t
, described above. If a color
is not provided to the stage definition, Quetoo automatically uses the surface's average static lighting color to shade the environment map.
{
material office/glass
{
envmap office/glass_envmap
blend GL_SRC_ALPHA GL_ONE
color 0.9 0.9 1.0
}
}
Lightmap stages are used to blend in statically computed lighting information. This is typically applied as the last drawn stage, and is useful for shading opaque or mostly opaque stages such as animations. Lightmap stages are declared by simply beginning the stage with the lightmap
type name. Lightmap stages do not support any unique directives.
{
material lunaran/computer0
...
{
anim 4 0.3
}
{
lightmap
}
}
Flare stages are used to add flares (coronas) to surfaces such as light fixtures. For convenience, flare stages may reference numeric values to take advantage of several built-in effect textures, e.g. flare 1
. Alternatively, a unique texture can also be provided, e.g. flare flares/my_flare
.
Flare stages do not currently support any unique directives, but do support color scale.s scale.t
described above. If a color
is not provided to the stage definition, Quetoo automatically uses the surface's average static lighting color to shade the flare. Either of the scale.s
or scale.t
directives can be used to influence the size of the flare produced by this stage.
{
material tech/small_light
...
{
flare tech/flare1
scale.s 1.5
}
}
The best way to learn the materials system is to study some of the materials files that come with Quetoo. These are conveniently available in the game data repository.